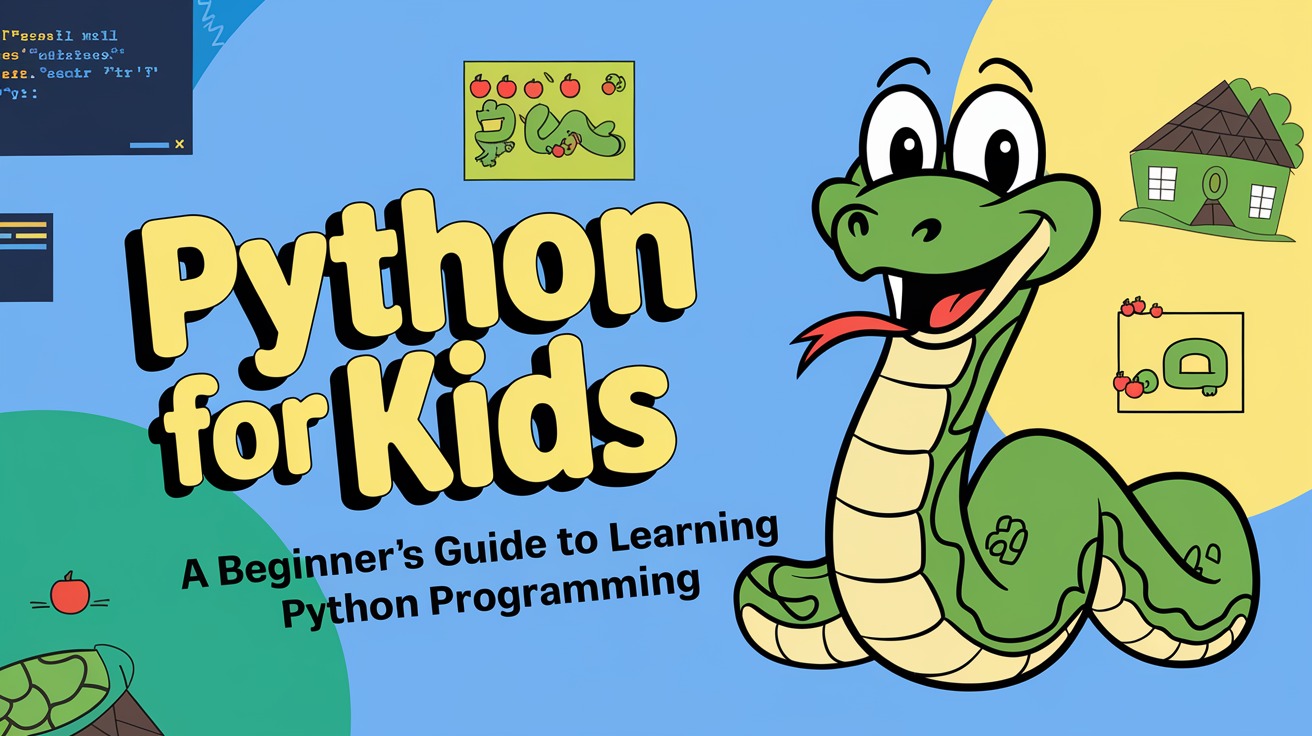
What is Python for Kids?
Python is a high-level, general-purpose programming language known for its simplicity and readability. For kids, Python is an ideal language because it uses plain English-like commands, making it easy to understand and apply. From creating games to building stories or analyzing data, Python offers endless possibilities for children to explore.
Kids can use Python for:
- Developing fun games like guessing games or simple quizzes.
- Drawing and designing shapes with Turtle Graphics.
- Automating repetitive tasks with basic programming logic.
- Exploring creative ideas such as interactive storytelling.
Python’s versatility means that kids can start small with simple programs and gradually move on to more advanced projects.
Why is Python Easy for Kids?
Python is considered one of the best programming languages for beginners, including kids, due to several factors:
- Simple Syntax: Python’s syntax is clean and straightforward, resembling everyday English. For example, printing text in Python is as simple as:
print("Hello, World!")
- This simplicity lowers the barrier to entry for young learners.
- Immediate Feedback: Kids can write code and see instant results, which keeps them motivated and curious to learn more.
- Community Support: Python boasts an extensive community filled with tutorials, forums, and resources tailored for beginners and kids.
- Versatility: Python is not limited to one domain—it can be used for games, web development, data analysis, and even art projects, allowing kids to pursue their interests.
How Learning Python Can Benefit Kids in the Long Run?
Learning Python at an early age has several advantages that extend beyond just coding:
- Builds Computational Thinking – Kids learn how to approach problems logically, analyze patterns, and develop step-by-step solutions.
- Improves Creativity – Python allows children to create unique projects, including games, animations, and interactive programs.
- Prepares for the Future – Technology is shaping the job market, and coding skills are becoming more important than ever.
- Boosts Academic Performance – Many studies suggest that learning to code improves problem-solving skills in subjects like mathematics and science.
- Encourages Persistence – Debugging errors and refining code teaches patience and resilience, skills that are valuable in all areas of life.
How to Teach Python to Kids?
Teaching Python to kids doesn’t have to be overwhelming. Here are some strategies to make the learning process engaging and effective:
- Start with Visual Tools: Tools like Scratch or Blockly introduce programming concepts visually before transitioning to text-based coding like Python.
- Leverage Interactive Libraries: Python’s Turtle Graphics library is a fantastic way to engage kids by allowing them to draw shapes and patterns with code.
Example:
import turtle
turtle.forward(100)
turtle.right(90)
turtle.circle(50)
Use Game-Based Platforms: Platforms like CodeCombat teach Python through interactive games, making learning both fun and immersive.
Focus on Hands-On Projects: Encourage kids to build small projects like a calculator, guessing games, or simple art programs to apply what they’ve learned.
Encourage Questions and Experimentation: Kids learn best when they’re curious. Let them experiment, make mistakes, and ask questions to deepen their understanding.
For a comprehensive guide read:
How to Teach Python to Kids?
Fundamentals of Python for Kids
Before kids start building fun projects with Python, they need to understand its fundamental concepts. Python is a simple yet powerful language that allows young learners to write code with minimal complexity. The fundamentals include variables, data types, operators, control flow (if-else statements and loops), functions, and basic data structures like lists, tuples, and dictionaries. These concepts form the foundation of programming and help kids develop problem-solving skills, logical thinking, and computational creativity. Learning these basics in an engaging way ensures that kids can move on to more advanced topics like game development, animations, and automation with confidence.
Understanding Variables and Data Types
In Python, variables store information. Think of a variable as a container that holds different types of data.
Common Data Types in Python
Strings (str) – Text data like "Hello" or "Python"Integers (int) – Whole numbers like 10, 200, -5Floats (float) – Decimal numbers like 3.14, 5.6Booleans (bool) – True or False, used for logicExample of Variables in Python
name = "Alice" # String
age = 12 # Integer
height = 4.8 # Float
is_student = True # Boolean
print(name, age, height, is_student)
In Python, you don’t need to declare the type of a variable; Python figures it out for you!
Introduction to Operators (Arithmetic, Logical, and Comparison)
Operators are symbols that perform calculations or comparisons in Python.
1. Arithmetic Operators
Used for basic math operations.
a = 10
b = 5
print(a + b) # Addition -> 15
print(a - b) # Subtraction -> 5
print(a * b) # Multiplication -> 50
print(a / b) # Division -> 2.0
print(a % b) # Modulus (remainder) -> 0
print(a ** b) # Exponentiation (power) -> 100000
2. Comparison Operators
Used to compare values.
x = 8
y = 12
print(x > y) # False
print(x == y) # False
print(x <= y) # True
3. Logical Operators
Used to make decisions in programs.
a = True
b = False
print(a and b) # False
print(a or b) # True
print(not a) # False
For a detailed guide about operators read: Operators In Python
Understanding Input and Output in Python
Python allows users to input values and output results.
1. Getting Input from Users
name = input("What is your name? ")
print("Hello, " + name + "!")
Here, input() takes user input, and print() displays the output.
2. Printing Multiple Values
age = 12
print("I am", age, "years old.")
Control Flow in Python for Kids
Control flow allows a program to make decisions and repeat actions.
What are Conditionals? (If-Else Statements)
Conditionals allow programs to respond differently based on conditions.
age = int(input("Enter your age: "))
if age >= 18:
print("You can vote!")
else:
print("You cannot vote yet.")
Here, if checks a condition, and else runs when the condition is false.
Understanding Loops (For and While Loops) with Examples
Loops let us repeat tasks automatically.
1. For Loop
Used when we know how many times to run.
for i in range(5):
print("Hello!") # Prints "Hello!" 5 times
2. While Loop
Runs as long as a condition is true.
x = 0
while x < 3:
print("Looping...")
x += 1 # Increases x by 1 each time
How to Use Nested Loops for More Complex Tasks
A nested loop is a loop inside another loop.
for i in range(3):
for j in range(2):
print(f"i: {i}, j: {j}")
This will print all combinations of i and j.
For a detailed guide read: Loops in Python for Kids
Python Functions for Kids
Functions organize code into reusable blocks.
What are Functions and Why Do We Need Them?
Functions help avoid repeating code and make programs easy to manage.
How to Create and Call Functions in Python
def greet():
print("Hello, welcome to Python!")
greet() # Calling the function
Using Functions to Make Code Reusable
def add_numbers(a, b):
return a + b
result = add_numbers(3, 5)
print(result) # Output: 8
For a detailed guide read: Functions in Python for Kids
Python Data Structures for Kids
Data structures store and organize data efficiently.
1. Introduction to Lists and How to Use Them
Lists store multiple items.
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # apple
2. Understanding Tuples and How They Differ from Lists
Tuples are like lists but unchangeable.
colors = ("red", "blue", "green")
3. What are Dictionaries and How to Use Them in Python?
Dictionaries store data in key-value pairs.
student = {"name": "Alice", "age": 12}
print(student["name"]) # Alice
Python Graphics and Animation for Kids
Introduction to Turtle Graphics: Making Art with Code
Turtle Graphics lets kids draw with Python.
import turtle
turtle.forward(100) # Moves forward by 100 pixels
Drawing Geometric Shapes Using Python Turtle
import turtle
for _ in range(4):
turtle.forward(100)
turtle.right(90) # Draws a square
Creating Fun Animations and Patterns
Kids can create spirals, flowers, and animations by looping movements.
For a detailed guide about animations read: Python Animations for Kids
Python Game Development for Kids
How to Build Simple Games in Python Using Pygame?
Pygame is a library for building interactive games.
Creating a Rock-Paper-Scissors Game with Python
import random
choices = ["rock", "paper", "scissors"]
computer = random.choice(choices)
player = input("Choose rock, paper, or scissors: ")
if player == computer:
print("It's a tie!")
elif (player == "rock" and computer == "scissors") or \
(player == "paper" and computer == "rock") or \
(player == "scissors" and computer == "paper"):
print("You win!")
else:
print("Computer wins!")
Fun Python Projects for Kids
Creating a Virtual Dice Roller
import random
print("You rolled:", random.randint(1, 6))
Building a Simple Chatbot with Python
def chatbot():
user_input = input("How can I help? ")
print("Sorry, I'm still learning!")
chatbot()
To get more Python coding ideas for kids read: 20+ Python Games and Fun Projects for Kids
How to Start Python Programming for Kids?
Getting started with Python programming for kids is easy and can be done in a few steps:
Step 1: Install Python and an IDE
How to Set Up Python on Your Computer?
- Download Python from the official website (python.org) and install the latest version.
- Install Python by following the guided instructions for your operating system.
- Open the Python Shell or an IDE to start coding.
- Test Installation by running
print("Hello, Python!")
in the terminal.
What is an IDE? Best Python IDEs for Kids
An IDE (Integrated Development Environment) tool allows kids to write, test, and debug their code.
The best IDEs for kids are:
Thonny – A simple and beginner-friendly interface designed for young learners.
VS Code – Ideal for kids who want a more advanced coding environment with additional features.
PyCharm Edu – Includes built-in lessons and an interactive learning interface.
For Selecting the best Python ide for your kid Read: 5 Best Python IDEs for Kids
Step 2: Learn Basic Commands
Introduce simple commands like:
Printing text: print("Hello!")
Using variables: name = "Alice"
Basic math: result = 5 + 3
Step 3: Practice with Loops and Conditionals
Loops and conditionals are fundamental to programming. Teach kids concepts like:
For Loop:
for i in range(5):
print("This is loop", i)
If Statement:
age = 10
if age > 8:
print("You are older than 8!")
Step 4: Build Fun Projects
Start with simple projects like a guessing game, drawing patterns with Turtle, or creating a text-based adventure game.
List 0f 30+ Fun Python Projects for Kids
Step 5: Encourage Regular Practice
Consistent practice is key. Provide kids with coding challenges or encourage them to explore new project ideas.
What are Basic Python Programs for School Students?
School students can create various basic programs to apply their Python knowledge. Here are a few examples:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
print("The sum is:", num1 + num2)
Guess the Number Game:
import random
number = random.randint(1, 10)
guess = int(input("Guess a number between 1 and 10: "))
if guess == number:
print("You guessed it!")
else:
print("Try again!")
To Know more Read: 30+ Python Coding Projects for High School Students
Turtle Graphics: Teach kids to draw shapes like squares, triangles, and circles using the Turtle library.
Text-Based Adventure Game: Let kids create a simple game where players make choices to navigate a story. This introduces conditionals and functions.
Why Use VS Code for Python Beginners?
Visual Studio Code (VS Code) is a lightweight yet powerful IDE that is perfect for Python beginners, including kids. Here’s why:
- User-Friendly Interface: VS Code is intuitive and easy to navigate, making it accessible for young learners.
- Extensions for Python: Install Python-specific extensions for auto-completion, debugging, and linting to simplify coding.
- Integrated Terminal: Kids can write and execute their code in the same window, reducing confusion.
- Cross-Platform Compatibility: VS Code works on Windows, macOS, and Linux, ensuring flexibility.
- Customizable Environment: Themes and plugins make the learning environment fun and tailored to individual preferences.
What is the Best Way to Learn Python for Class 6 to Class 9?
Python For Class 6 Students:
- Use Visual Tools: Start with Python Turtle Graphics to draw fun patterns and introduce loops and variables.
- Relate to Interests: Incorporate games, stories, or art projects to keep learning engaging.
- Practice Small Projects: Examples include making a name banner or a number guessing game.
Python For Class 7 Students:
- Dive Deeper into Loops and Conditionals: Teach while introducing more complex loops and conditional statements. Use examples like “If-Else” statements in real-world scenarios.
- Explore Lists and Dictionaries: Introduce data structures like lists and dictionaries, helping kids manage data more efficiently.
- Build Mini Games: Develop simple games like rock-paper-scissors or a quiz game, which enhance problem-solving and logical thinking.
Python For Class 8 Students:
- Introduce Functions: Teach the importance of functions in programming. Show how functions help in making code more reusable and organised.
- Hands-On Projects: Encourage small projects like a contact book, a calculator, or a to-do list application to apply Python concepts.
- Introduce Libraries: Start exploring libraries like random and time to build more interactive and dynamic programs.
Python For Class 9 Students:
- Introduce Real-World Applications: Teach data handling, automation, and web scraping using libraries like Pandas or Beautiful Soup.
- Encourage Project-Based Learning: Assign tasks like creating a quiz app, a personal diary program, or analyzing data sets.
- Explore Advanced Concepts: Introduce functions, classes, and file handling to deepen their understanding.
Python For Class 10 Students:
- Advanced Data Structures: Introduce more complex data structures like sets, tuples, and multi-dimensional lists. Encourage solving problems that involve these structures.
- Introduction to Web Development: Teach the basics of web development using Python frameworks like Flask or Django. Let students build basic web apps like a portfolio website.
- Problem-Solving Challenges: Push students to engage in coding challenges from platforms like HackerRank or Codewars to sharpen their skills and apply Python in algorithmic problem-solving.
Ready to Get Started?
Python provides an excellent gateway for kids to explore the world of programming, combining creativity with problem-solving. If you’re looking for a structured and fun way to introduce Python to your kids, check out the Python Basics for Kids Course by Modern Age Coders. This course simplifies coding with interactive lessons, engaging projects, and hands-on support. Perfect for beginners, it’s the ideal stepping stone into coding.
Conclusion
Python is an excellent programming language for kids due to its simplicity, versatility, and vast resources. By starting with visual tools, building small projects, and gradually exploring advanced concepts, kids can unlock their creative potential and develop essential coding skills. Whether it’s drawing with Turtle Graphics, creating games, or automating tasks, Python makes coding accessible and enjoyable for learners of all ages.